(Not) looping over lists in PHP
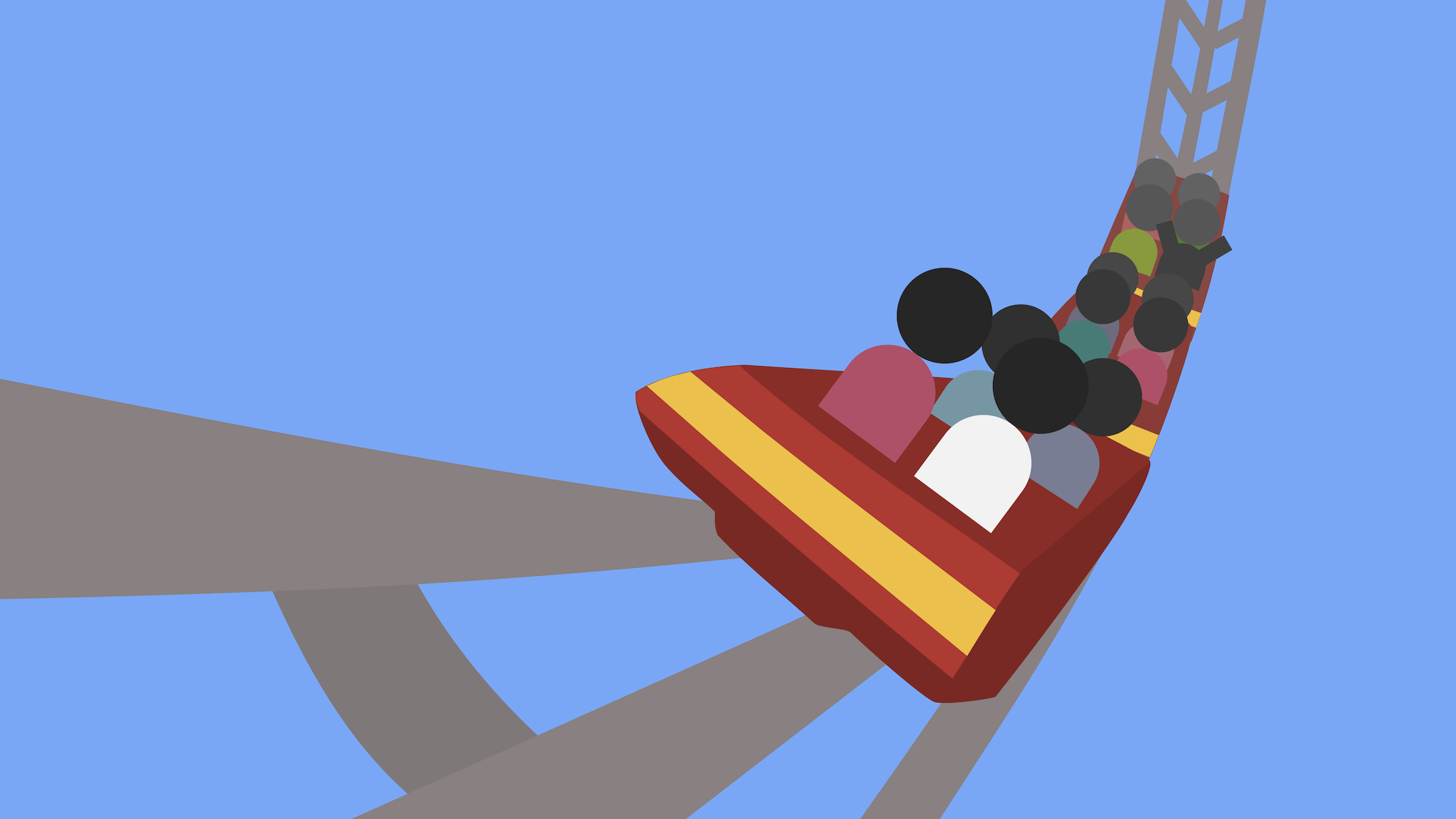
This is a lazy blog post for people who are too lazy to browse through
PHP’s array function documentation.
I’ll show three methods that help you create new lists or values based on an
existing list: array_map()
, array_reduce()
, and array_filter()
.
Let’s say we have a list of numbers that we want to multiply by 2:
We can use array_map()
to map (=convert) all values in this
list:
Note that array_map()
does not modify the original list – instead it returns a
new list that contains the converted values:
Here’s the corresponding looping version:
I used an anonymous function in the example above and will keep doing that for the sake of brevity in the remainder of this article. But you can always use other types of functions if you want, like…
Named functions:
Static methods:
Or instance methods:
Let’s assume we have a list of $numbers
again, but this time we want to
reduce it to a single aggregate value, like a sum:
Sounds like a nice job for
array_reduce()
!
Like array_map()
, this calls a user-supplied function for each element. There
are some important differences however:
-
array_map()
expects the function as the first and the array as the second argument.array_reduce()
on the other hand, expects the ; -
Your function now expects two arguments:
$carry
and$item
.$carry
is a variable that “carries” the intermediate aggregation result. In this case, it’s a number that’s used to keep track of the sum; -
The third argument to
array_reduce()
can be used to initialise$carry
. It’s technically optional, but I would recommend that you always provide it.
We can verify that our $sum
is correct by comparing it with the output of
array_sum()
, which does the same in less lines of code:
The iterative version is much simpler, which is probably why you don’t see
array_reduce()
“in the wild” very often.
Nevertheless, it’s a pretty powerful function that can be used to implement more high-level array functions.
For instance, you can use it to check if a list only contains true values:
Or make sure that a list contains at least one true value:
Suppose that we still have that same list of $numbers
, but this time we only
want to filter (=keep) odd values:
We can use array_filter()
to create a new array that only
contains values that we want to keep:
Here’s the looping version of that same code:
As you can see, $oddNumbers
will now be an associative array!
This will cause issues when you serialise it into JSON. The result will not be a list, but an object with numeric keys:
You can easily fix this using array_values()
, which creates a new, re-indexed
version of your array:
Read PHP’s array documentation if you want to learn more about the other seventy-something array functions!
Use…
-
array_map()
to convert all values in a list -
array_reduce()
to turn a complete list into a single value -
array_filter()
to only keep certain elements